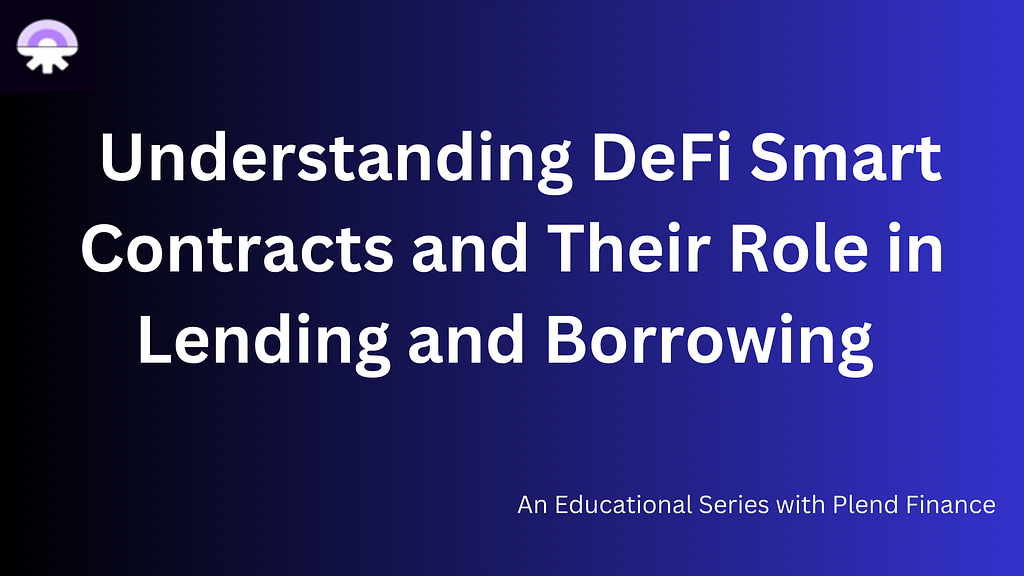
Plenty :: Lending and Borrowing in DeFi 2: Understanding DeFi Smart Contracts and Their Role in Lending and…
July 12, 2024Lending and Borrowing in DeFi 2: Understanding DeFi Smart Contracts and Their Role in Lending and Borrowing
In the first episode, we explored the basics of lending and borrowing in DeFi, highlighting the benefits, key platforms, and the overall process. In this episode, we’ll dive deeper into the backbone of DeFi: smart contracts. We’ll discuss what smart contracts are, how they function, and their pivotal role in enabling secure and transparent lending and borrowing in the DeFi ecosystem.
What are Smart Contracts?
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They run on supported blockchain networks, with the most contracts being written and executed on Ethereum. Now, understand that in a smart contract, the terms are executed only when predefined conditions are met.
Learn more about smart contracts here
Key Features of Smart Contracts
Automated Execution: Smart contracts eliminate the need for intermediaries by automating the execution of agreements when conditions are met.Transparency: All smart contract transactions are recorded on the blockchain, ensuring complete transparency and traceability.Immutability: Once deployed, smart contracts cannot be altered, which guarantees the integrity and security of the terms.Trustlessness: Parties involved do not need to trust each other; they only need to trust the code.
How Smart Contracts Enable Lending and Borrowing in DeFi
Smart contracts are fundamental to DeFi lending and borrowing platforms like Plend, Aave, and Compound. Here’s how they work:
Collateral Management:Users deposit their crypto assets (e.g., $DAI) into a smart contract as collateral.The smart contract locks the collateral and verifies its value.
2. Loan Issuance:
Once the collateral is secured, the smart contract facilitates the borrowing of assets (e.g., $ETH).The terms of the loan, including interest rates and repayment schedules, liquidation threshold, loan-to-value ratio(LTV),etc are encoded in the smart contract.
3. Interest and Repayments:
Borrowers repay the loan along with interest directly to the smart contract.The smart contract ensures that lenders receive their interest payments on time.
4. Liquidation:
If the value of the collateral falls below a certain threshold, the smart contract automatically triggers liquidation.The collateral is sold to repay the loan, protecting lenders from default risk.
Example Scenario:
User A wants to borrow ETH from PlendUser A deposits DAI as collateral into a smart contract on Plend.The smart contract locks the DAI and allows User A to borrow ETH.User A repays the ETH loan with interest over time.If the DAI collateral’s value drops too low, the smart contract liquidates it to repay the ETH loan.
For a more technical rundown of key details in a basic smart contract designed for a lending protocol, see below ⬇️
PS: If you are not technical, you can choose to skip this part 🙂
(a) Imports and Libraries
This helps to manage dependencies and use the smart contract’s code more efficiently.
import “@openzeppelin/contracts/utils/math/SafeMath.sol”;
import “@openzeppelin/contracts/token/ERC20/IERC20.sol”;SafeMath: Prevents overflow and underflow errors in arithmetic operations.IERC20: Interface for interacting with ERC20 tokens (e.g., DAI).
(b) Contract Variables
IERC20 public stablecoin;
uint256 public collateralizationRatio = 150;
uint256 public interestRate = 10;stablecoin: The ERC20 stablecoin used for borrowing (e.g., DAI).collateralizationRatio: The required collateral ratio (e.g., 150%).interestRate: Annual interest rate for borrowing.
(c) Loan Struct
struct Loan {
uint256 collateral;
uint256 debt;
uint256 timestamp;
}collateral: Amount of ETH deposited as collateral.debt: Amount of stablecoin borrowed.timestamp: Time when the loan was created.
(d) Mappings and Events
mapping(address => Loan) public loans;
event Deposited(address indexed user, uint256 amount);
event Borrowed(address indexed user, uint256 amount);
event Repaid(address indexed user, uint256 amount);
event Withdrawn(address indexed user, uint256 amount);loans: Maps user addresses to their loan details.Events: Log deposit, borrow, repay, and withdraw actions.
(e) Constructor
Initializes the contract with the stablecoin address.
constructor(address _stablecoin) {
stablecoin = IERC20(_stablecoin);
}
(f) Deposit Collateral Function
Allows users to deposit ETH as collateral.
function depositCollateral() external payable {
require(msg.value > 0, “Collateral amount must be greater than 0”);
Loan storage loan = loans[msg.sender];
loan.collateral = loan.collateral.add(msg.value);
emit Deposited(msg.sender, msg.value);
}Allows users to deposit ETH as collateral.
(g) Borrow Function
Allows users to borrow stablecoins against their collateral.
function borrow(uint256 amount) external {
Loan storage loan = loans[msg.sender];
uint256 maxBorrow = loan.collateral.mul(100).div(collateralizationRatio);
require(amount <= maxBorrow, “Borrow amount exceeds collateralization ratio”);
loan.debt = loan.debt.add(amount);
loan.timestamp = block.timestamp;
require(stablecoin.transfer(msg.sender, amount), “Stablecoin transfer failed”);
emit Borrowed(msg.sender, amount);
}
(h) Repay Function
Allows users to repay their borrowed stablecoins.
function repay(uint256 amount) external { Loan storage loan = loans[msg.sender]; require(amount <= loan.debt, “Repay amount exceeds debt”); loan.debt = loan.debt.sub(amount); require(stablecoin.transferFrom(msg.sender, address(this), amount), “Stablecoin transfer failed”); emit Repaid(msg.sender, amount); }
(i) Withdraw Collateral Function
Allows users to withdraw their collateral after repaying their debt.
function withdrawCollateral(uint256 amount) external { Loan storage loan = loans[msg.sender]; require(loan.debt == 0, “Debt must be repaid before withdrawing collateral”); require(amount <= loan.collateral, “Withdraw amount exceeds collateral”); loan.collateral = loan.collateral.sub(amount); payable(msg.sender).transfer(amount); emit Withdrawn(msg.sender, amount); }
(j) Interest Calculation Function
Calculates the interest on the debt over a given duration.
function calculateInterest(uint256 debt, uint256 duration) public view returns (uint256) { return debt.mul(interestRate).mul(duration).div(365 days).div(100); }
(k) Get Loan Details Function
Returns the loan details for a given user.
function getLoanDetails(address user) external view returns (uint256, uint256, uint256) { Loan storage loan = loans[user]; return (loan.collateral, loan.debt, loan.timestamp); }
Usage
Deploy the Contract: Deploy the contract on a blockchain network (e.g., Ethereum) with the address of the stablecoin (e.g., DAI).Deposit Collateral: Users can deposit ETH as collateral.Borrow: Users can borrow stablecoins against their deposited collateral.Repay: Users can repay their borrowed stablecoins.Withdraw Collateral: Users can withdraw their collateral after repaying their debt.
This is just a basic implementation or usage of the smart contract code above, you can easily use this or even try to test it out. But in a real case scenario, you’d want to do more research, add more features, vet the code and add security checks to make it more robust and secure.
Advantages of Smart Contracts in DeFi
Efficiency: Automated processes reduce the time and cost associated with traditional financial intermediaries.Security: Code-enforced rules and immutability provide a high level of security.Global Access: Anyone with an internet connection can participate in DeFi, promoting financial inclusion.Innovation: Smart contracts enable the creation of complex financial products and services without the need for centralized institutions.
Challenges and Risks
Smart Contract Bugs: Errors in a code can lead to vulnerabilities and potential losses, especially when deployed on a main network.Market Volatility: Fluctuations in crypto asset values can affect collateral and loan stability. This makes it very important to monitor your assets and mitigate risk of liquidation.Regulatory Uncertainty: The evolving regulatory landscape may impact DeFi operations as regulations are unknown and not clear to users yet.
Future Trends in Smart Contracts
Cross-Chain Interoperability: Plend is at the top of this innovation as we are enhancing smart contract functionality so users can move liquidity across different blockchains seamlessly.Improved Security Audits: More rigorous auditing practices to minimize bugs and vulnerabilities.Enhanced User Interfaces: Simplifying interaction with smart contracts for non-technical users.
Conclusion
Smart contracts are the cornerstone of DeFi, enabling secure, transparent, and efficient lending and borrowing. By automating processes and eliminating intermediaries, they unlock new possibilities for financial innovation and inclusion.
At Plend, we are focused on eliminating the issues that users face while interacting with native DeFi protocols such as smart contract and economic risks, coupled with liquidity fragmentation.
Try out the Plend testnet here.
Make sure to follow on X to get the latest development updates
Join the community on discord 💜🫂